Integrate Deepseek into Salesforce with GPTfy
Table of Contents
TL;DR:
Microsoft announced DeepSeek R1 on Azure. Within hours, our team connected it to Salesforce using GPTfy’s no-code declarative configuration. This guide covers Named Credential setup, security configurations, Apex interfaces for flexibility, and prompt engineering optimized for DeepSeek. The blueprint is suitable for any organization, allowing deployment of this enterprise-grade architecture without specialized AI expertise.
Not a fan of reading articles? Check out the video here:
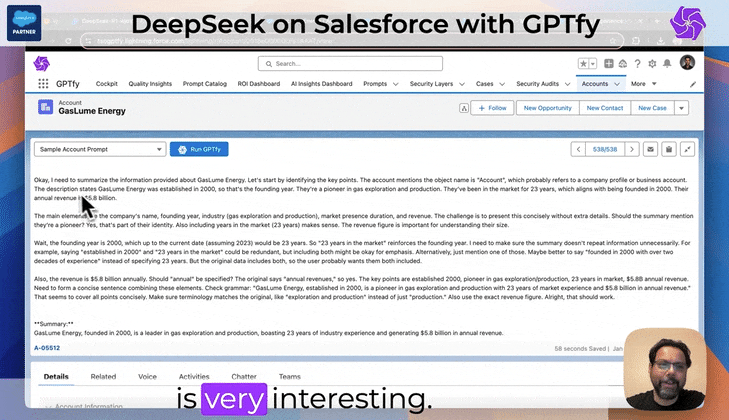
What?
A practical implementation guide for connecting Microsoft Azure’s DeepSeek R1 reasoning model to your Salesforce environment through GPTfy’s declarative architecture – complete with security guardrails and enterprise integration patterns.
Who?
Salesforce admins, architects, developers, and technical leaders who need to deploy AI capabilities quickly while maintaining security, compliance, and architectural flexibility.
Why?
To leverage DeepSeek’s superior reasoning capabilities without the typical 3-6 month AI implementation timeline, while future-proofing your investment against the rapid evolution of AI models.
→ Deploy day-one technologies today. Protect sensitive data. Maintain flexibility for tomorrow.
What can you do with it?
Automated Code Analysis: Instantly check Apex classes against organizational standards
Standardized Quality Gates: Ensure consistent enforcement of coding policies
Developer Guidance: Provide specific feedback with examples for improvement
Technical Debt Management: Track code quality evolution over time
Technical Architecture Overview
Let’s cut through the complexity and focus on what matters – a secure, adaptable architecture that connects Salesforce to DeepSeek without unnecessary overhead:
The integration follows a modular pattern with five key components:
- Named Credential Layer: Securely manages authentication without exposing credentials
- GPTfy Processing Framework: Handles data extraction and formatting
- Prompt Engineering Framework: Structures communication for optimal results
This approach provides enterprise-grade capabilities without the enterprise-grade complexity typically associated with AI implementations.
1. Declarative DeepSeek Configuration
DeepSeek R1 setup uses Salesforce’s native Named Credential pattern – no custom authentication code or complex integrations required.
Named Credential Configuration
Label: Azure DeepSeek
Name: AzureDeepSeek
URL: https://[your-azure-instance].openai.azure.com/openai/deployments/DeepSeek-R1/chat/completions?api-version=2024-02-15-preview
Authentication: Named Principal
Authentication Protocol: OAuth 2.0
Authentication Provider: Azure OpenAI
This follows Salesforce’s security best practices, keeping sensitive credentials managed by the platform rather than embedded in code.
AI Model Configuration in GPTfy
The AI model configuration is straightforward but powerful:
Model Name: DeepSeek R1
Platform: Azure OpenAI
Named Credential: AzureDeepSeek
AI Technology: DeepSeek
Type: Chat
Temperature: 0.1 (configurable)
Top P: 0.10 (configurable)
Max Tokens: 2000 (configurable)
These parameters control DeepSeek’s reasoning process – lower temperature values produce more deterministic responses, while higher values allow for more creative exploration.
Implementation Example
Rather than wading through complex configuration, you can set this up through GPTfy’s intuitive interface:
- Access the GPTfy Cockpit → AI Models → Create Your Own
- Fill in model details, pointing to your Azure instance
- Save and activate
- Test on a sample record
The entire configuration process takes less than 30 minutes – no deployment packages, no code reviews, no lengthy testing cycles.
2. Enterprise-Grade Security Implementation
Security isn’t an afterthought – it’s built into the core architecture through a multi-layered approach that’s both comprehensive and pragmatic.
Layer 1: Field-Level Protection
This layer focuses on explicit field values containing sensitive information:
Field: Email
Protection Type: Entire Value
When email value john.doe@example.com is processed, it’s automatically replaced with a secure token like [SF-0123-001] before reaching DeepSeek.
Layer 2: Regex-Based Pattern Detection
This intelligent layer identifies and masks sensitive patterns within text fields:
// Sample regex pattern configuration
Pattern Name: US Phone Number
Pattern: \(?\d{3}\)?[-.\s]?\d{3}[-.\s]?\d{4}
Match Complete Word: true
Ignore Special Characters: true
For example, if “Call me at (555) 123-4567 to discuss” appears in a description field, the phone number is automatically masked before sending to DeepSeek.
Layer 3: Global Blocklist Protection
The third security layer handles specific terms you never want sent to external AI models:
Blocklist Category: Product Names
Values: [Confidential Product X, Secret Project Y, Unreleased Feature Z]
Every interaction creates a comprehensive security audit record for compliance and monitoring:
This approach ensures DeepSeek never sees sensitive information while maintaining context and utility in responses – giving you both security and functionality without compromise.
3. Advanced Prompt Engineering for DeepSeek
DeepSeek R1 excels at reasoning tasks, making prompt engineering crucial for optimal results. Here’s what works:
Basic DeepSeek Account Analysis Prompt
Title: Sales Opportunity Recommendation Engine - Activity-Based Analysis
IMPORTANT: Generate a comprehensive sales opportunity analysis with accurate activity counts, engagement metrics, and detailed recommendation analysis.
Input Structure:
The input will be a JSON object containing:
- Task: Array of task activities
- Event: Array of event activities
- Recommendations__r: Array of product recommendations
- Contacts: Array of stakeholder information
- Description: Account overview and challenges
- Name: Account name
- Industry: Account industry
Step 1: Data Processing
Calculate the following metrics:
1. Total Activities MUST be calculated as follows:
- Task count = Number of items in Task array (if exists, else 0)
- Event count = Number of items in Event array (if exists, else 0)
- Total Activities = Task count + Event count
- Example:
* If Task has 1 item and Event has 1 item, Total = 2
* If only Task has 1 item, Total = 1
* If neither exists, Total = 0
- Important: ALWAYS count both Task and Event arrays separately and sum them
2. Engagement Score (0-10 scale):
- Base score: 5 points
- Activity bonus: 0.1 points per activity (sum of Tasks AND Events)
- Recommendation bonus: 1.25 points per recommendation
- Cap at 10 points
- Example:
* 2 activities (0.2 points) + 2 recommendations (2.5 points) + base (5) = 7.7
3. Stakeholder Analysis:
- Format: "${FirstName} ${LastName} - ${Title}"
- Include role-specific description
- Extract priorities from Event descriptions related to stakeholder
4. Focus Areas:
- Extract from stakeholder descriptions
- Include themes from Event descriptions
- Present as comma-separated list
- Prioritize recurring themes
5. Recommendation Analysis:
- Priority Score (0-100):
* Pain point alignment: 30%
* Stakeholder preference match: 25%
* Financial impact: 25%
* Implementation feasibility: 20%
- Key Benefits: List specific product advantages
- Stakeholder Alignment: Map benefits to stakeholder needs
Required Output Format:
Return the following HTML structure exactly as shown, without any markdown formatting or code block indicators:
Account Overview
Account Name: {{Name}}
Industry: {{Industry}}
Key Challenges: {{Description}}
Activity Analysis
Total Activities
{{ActivityCount}}
Engagement Score
{{EngagementScore}}
Key Focus Areas
{{FocusAreas}}
Stakeholder Insights
{{#each Contacts}}
{{FirstName}} {{LastName}} - {{Title}}
{{Description}}
{{/each}}
Recommended Solutions
{{#each Recommendations__r}}
{{Name}}
{{Product__r.Description}}
Est. Revenue: ${{formatNumber Estimated_Revenue__c}}
Est. Margin: ${{formatNumber Estimated_Margin__c}}
Key Benefits:
{{#each KeyBenefits}}
- {{this}}
{{/each}}
Stakeholder Alignment:
{{#each StakeholderAlignment}}
- {{this}}
{{/each}}
Priority Score: {{PriorityScore}}
{{/each}}
Data Processing Rules:
1. Activity Count (MUST FOLLOW EXACTLY):
- ALWAYS check both Task and Event arrays
- Task count = Task array length (if exists, else 0)
- Event count = Event array length (if exists, else 0)
- Total = Task count + Event count
- Validate that both arrays are counted before outputting total
2. Engagement Score:
- Calculate based on actual metrics
- Base: 5 points
- Activities: +0.1 per activity (sum of ALL Tasks AND Events)
- Recommendations: +1.25 per recommendation
- Round to one decimal place
- Maximum: 10 points
3. Currency Formatting:
- Add commas for thousands
- Show cents if present
- Include dollar sign
- Example: $1,234,567.89
4. Priority Score Calculation:
- Pain point alignment (30%): How well solution addresses key challenges
- Stakeholder preference match (25%): Alignment with stakeholder needs
- Financial impact (25%): Revenue and margin potential
- Implementation feasibility (20%): Ease of implementation
- Score should be 0-100, rounded to nearest integer
5. Focus Areas:
- Extract from Event descriptions if available
- Combine with themes from Contact descriptions
- Prioritize based on frequency of mention
- Present as comma-separated list
DO NOT:
- Add markdown code block indicators
- Include any HTML tags outside the template
- Modify the existing HTML/CSS structure
- Add numerical suffixes to names
- Use placeholder values
- Skip any analysis sections
- Change scoring weights
- Alter the template format
- Skip counting either Task or Event arrays
- Output activity count before checking both Task and Event arrays
Pre-Processing Validation:
1. Confirm presence of both Task and Event arrays
2. Count items in each array separately
3. Sum the counts before displaying total activities
4. Verify engagement score calculation includes all activities
5. Double-check currency formatting
6. Validate priority score calculations
The last line is particularly important – it leverages DeepSeek’s reasoning capabilities to provide transparency into how it reached its conclusions.
Grounding Rules Configuration
These guardrails ensure consistent, high-quality responses:
Ethical Grounding:
- Do not make assumptions about individuals based on demographics
- Present balanced perspectives
- Avoid using emotive language
Content Grounding:
- Provide your complete reasoning process
- Always state the objective evidence for your conclusions
- When referencing numbers, include the source data
- Avoid speculating about information not provided
Dynamic Grounding:
- When referencing dates and amounts, adhere to the USA locale
Implementation in Prompt Builder
All of this is configured through the GPTfy Prompt Builder – no code required:
- Create prompt with specific configuration
- Add grounding rules from the library
- Test with sample records
- Activate for production use
Bring Any AI Models to Your Salesforce
Handle questions securely with AI. Works with Pro, Enterprise & Unlimited - your data never leaves Salesforce.
Get GPTfyRead More hereReal-World Implementation
Let’s look at DeepSeek R1 in action with practical use cases you can implement right now:
Use Case: Data-Driven Sales Opportunity Analysis
In our testing environment, we implemented a “DeepSeek Test Prompt” for the GasLume Energy account to analyze complex sales opportunities with multiple stakeholders and engagement metrics.
The screenshot demonstrates DeepSeek’s reasoning capabilities in action:
What makes this implementation powerful is DeepSeek’s ability to show its complete reasoning process. This transparency builds trust with sales teams, who can verify the logic behind AI-driven recommendations.
The analysis continues with DeepSeek processing structured data to:
- Calculate engagement scores based on activity metrics
- Format stakeholder information from contacts
- Identify focus areas from descriptions and priorities
- Perform recommendation analysis with multi-criteria scoring
Beyond this demonstration, you can easily adapt this approach for:
- Opportunity Prioritization – Analyze pipeline deals to identify which ones deserve immediate attention based on engagement indicators and probability calculations
- Contract Risk Analysis – Scan agreements for compliance issues, unusual terms, and potential liabilities, with transparent reasoning for each flag
- Customer Support Enhancement – Generate technical solutions with step-by-step explanations, citing relevant knowledge base articles
- Executive Briefing Preparation – Create comprehensive account summaries with relationship history and strategic recommendations
The beauty of this architecture is that these use cases require no additional code or complex configuration – just focused prompt engineering that leverages DeepSeek’s reasoning capabilities.
Implementation Roadmap
Phase 1: Foundation (1-2 days)
- Configure Azure DeepSeek R1 deployment
- Set up Named Credentials in Salesforce
- Configure GPTfy AI Model
- Test basic connectivity
Phase 2: Security Framework (1 day)
- Configure data context mapping
- Set up security layers for PII protection
- Test with sensitive data records
Phase 3: Use Case Development (3-5 days)
- Develop initial prompts for your specific business needs
- Configure appropriate grounding rules
- Test and refine based on response quality
Phase 4: Production Deployment (1 week)
- Deploy to the production environment
- Train users on capabilities and limitations
- Establish a feedback loop for continuous improvement
This approach ensures you implement technology and create business value from day one.
Conclusion
Within hours of Microsoft announcing DeepSeek R1 on Azure, we had it integrated with Salesforce through GPTfy’s zero-code configuration – demonstrating what’s possible with the right architecture.
This isn’t just about speed – it’s about building a technical foundation that gives you immediate access to cutting-edge reasoning capabilities while maintaining enterprise-grade security and complete flexibility for whatever comes next in AI.
The multi-layered security system ensures sensitive data never leaves your Salesforce environment, while the model-agnostic approach lets you adapt as AI capabilities evolve. And with a structured prompt engineering framework, you can leverage DeepSeek’s superior reasoning abilities from day one.
The bottom line: You don’t need to choose between speed and quality when implementing AI. With this architecture, you can deploy cutting-edge capabilities in days, not months, while maintaining the security and flexibility your business requires.
Additional Resources
- Calculate Salesforce + AI ROI here
- Check out GPTfy Knowledge Base
- Check out other Salesforce + AI Use Cases
- Watch the Salesforce + AI Use Cases Demo
- Check out the GPTfy Blog for more blogs on Salesforce + AI
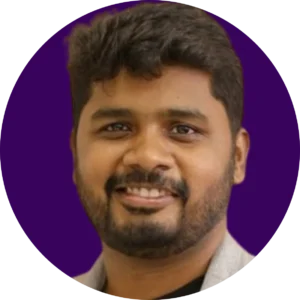
Kalanithi Balasubramanian
Kalanithi is a Senior Salesforce Engineer specializing in AppExchange solutions, AI-driven integrations, and workflow automation, with a track record of enhancing enterprise efficiency through LLM-powered innovations.
Blogs you may find interesting
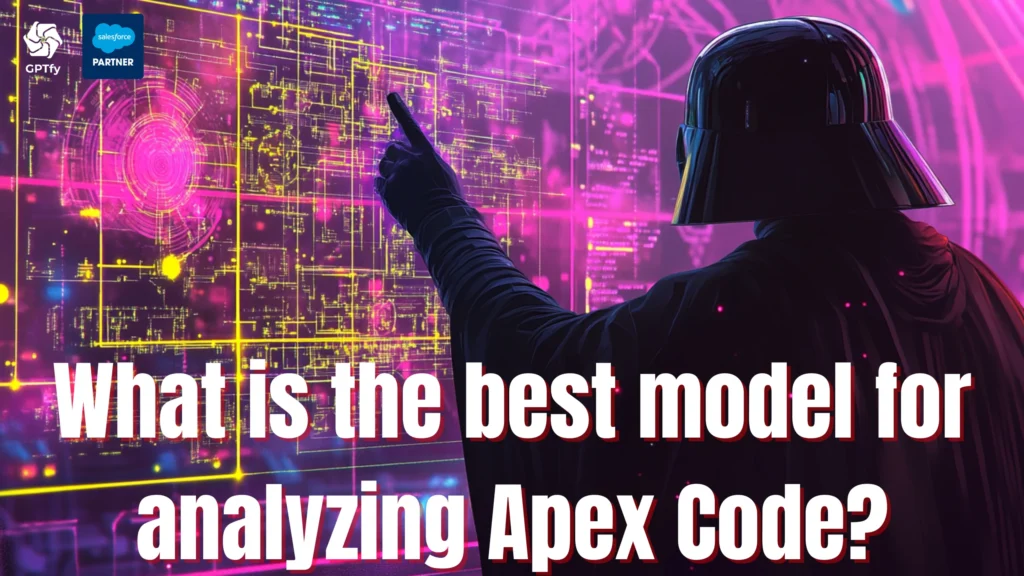
Comparing Top 3 AI Models for Salesforce Apex Code Reviews with GPTfy
See which AI models is best when it comes to Apex Code Review in Salesforce
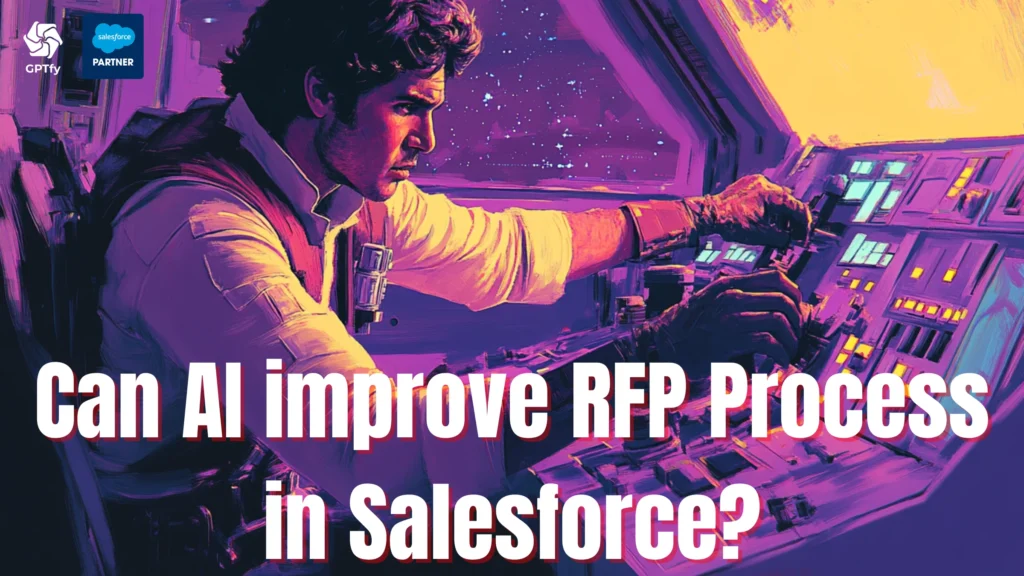
How to Improve Your RFP Process Using Salesforce + AI: A Practical Guide
See how AI can improve your RFP Process with AI in Salesforce
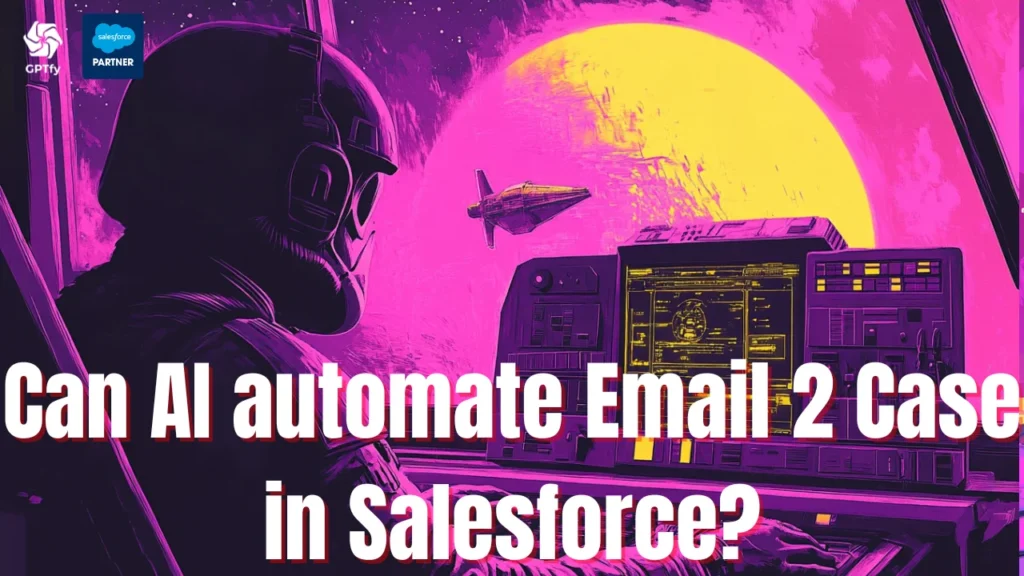
How to Automate Salesforce Email-to-Case with AI
See how AI can improve your Email 2 Case in Salesforce
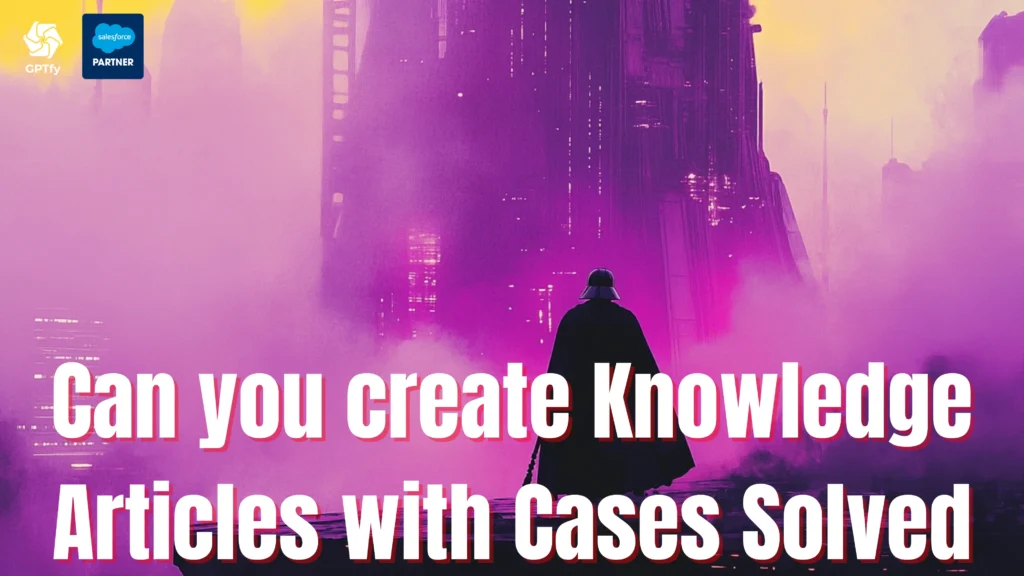
How to Create Knowledge Articles from Cases Solved in Salesforce?
See how AI can create Knowledge Articles from Cases Solved in Salesforce
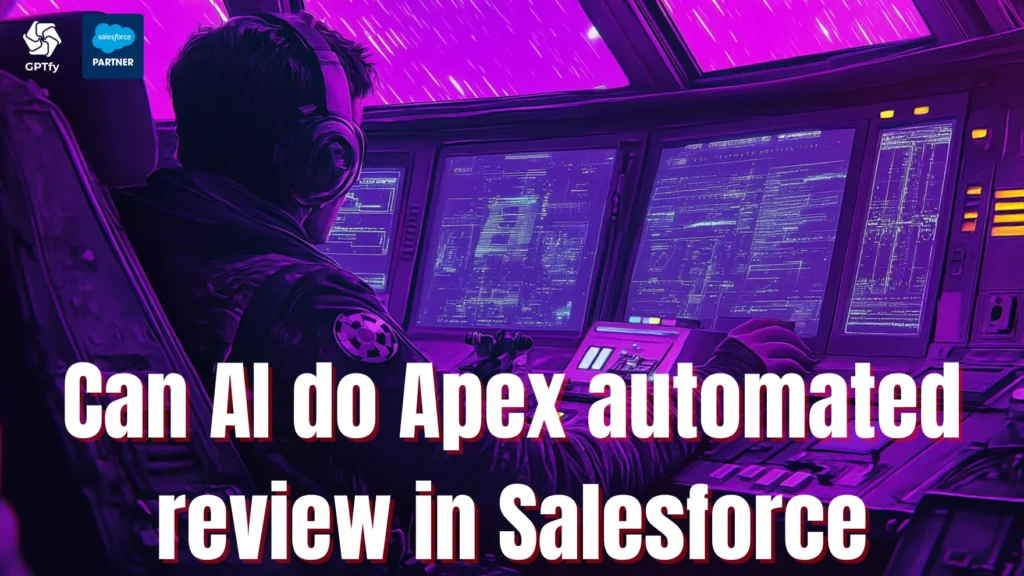
Automate Apex Code Reviews in Salesforce with AI using GPTfy
Learn how AI can do Apex Code Reviews in Salesforce
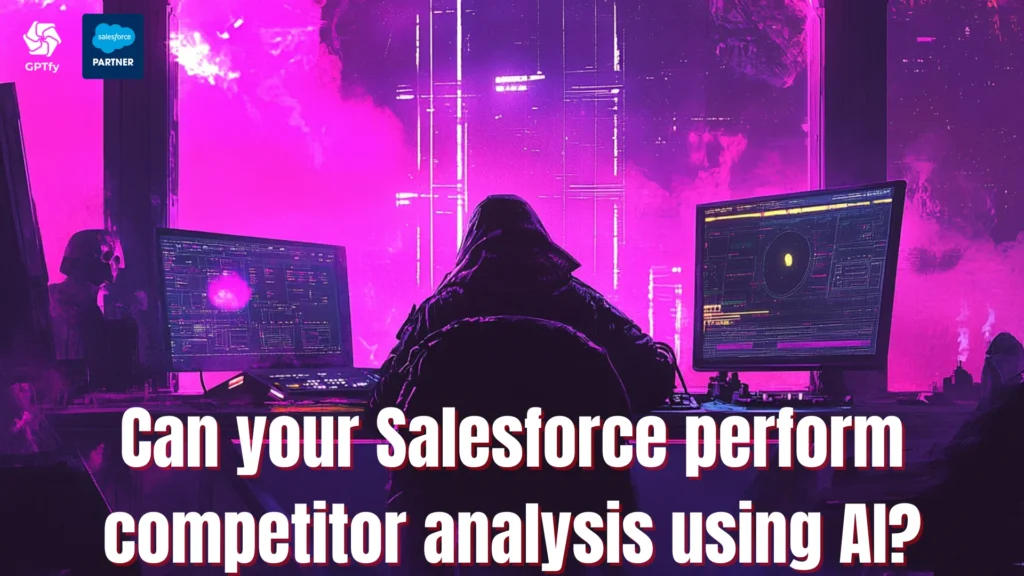
Utilizing AI for web scraping in Salesforce
Learn how AI can give you competitive insights inside Salesforce with GPTfy.
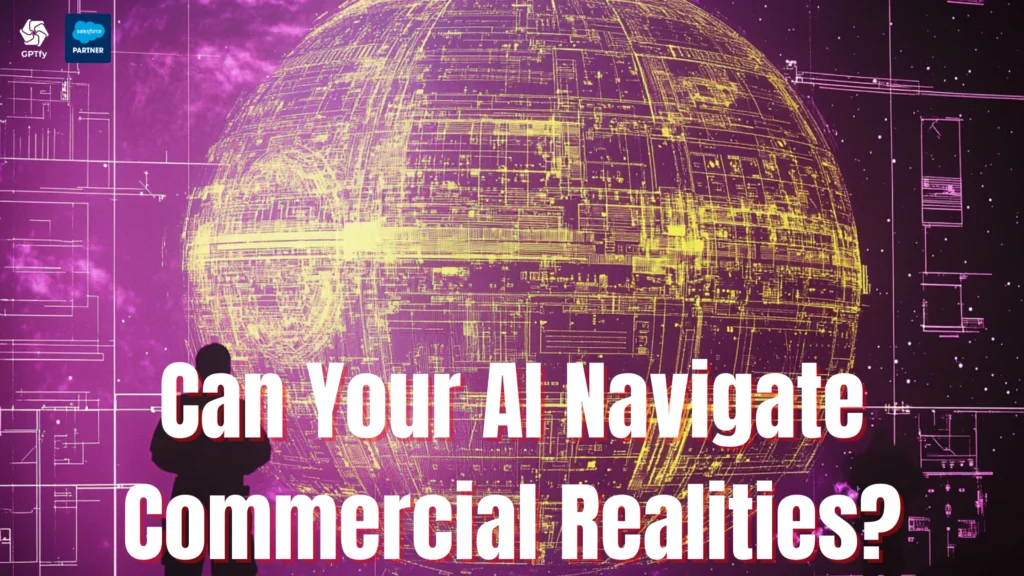
Navigating Commercial Realities & Internal Dynamics
Understand the key factors that can impact your Salesforce AI project
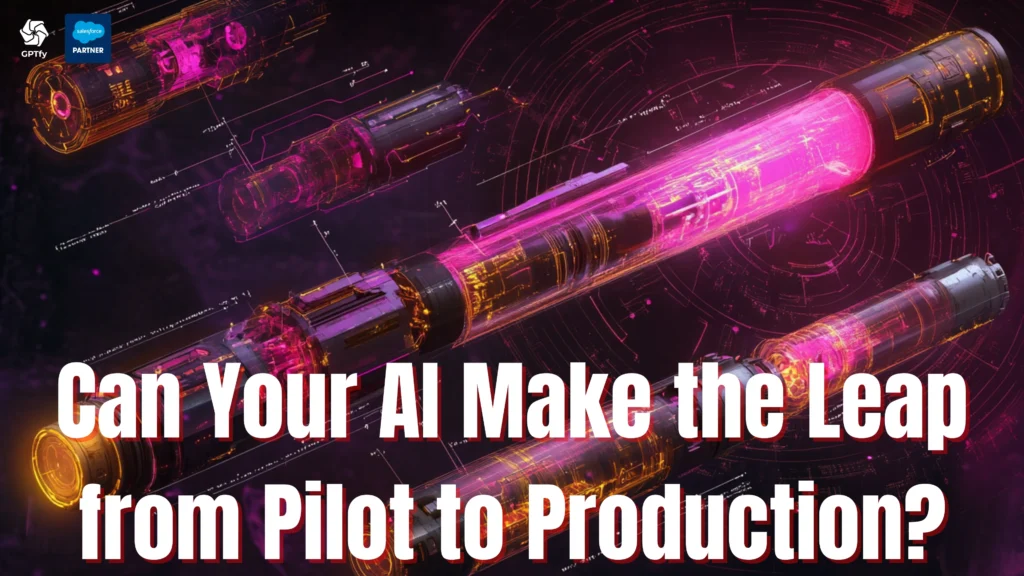
Making It Work – Salesforce + AI From Pilot to Production
Move from AI planning to real-world implementation in your Salesforce environment
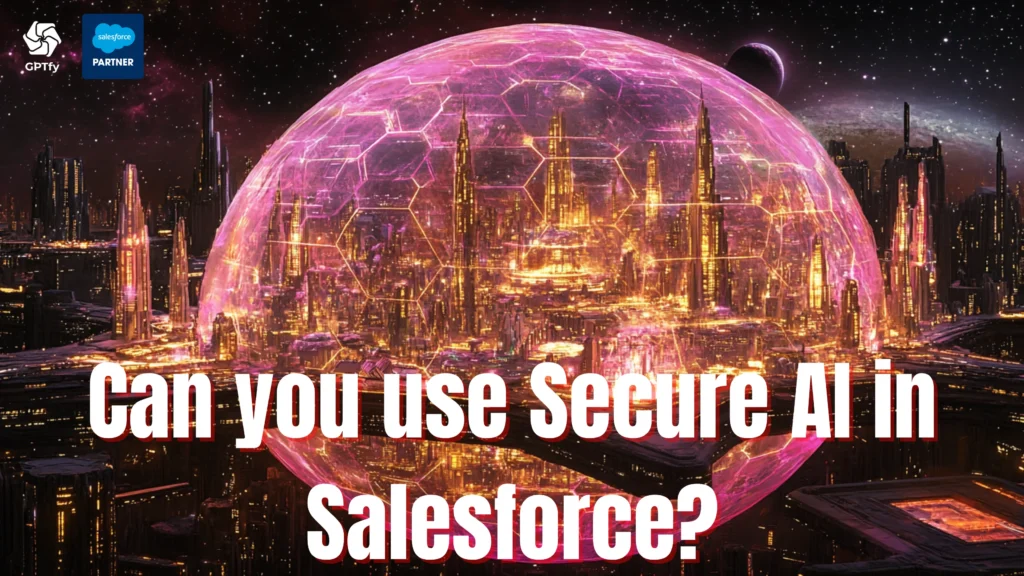
Keep AI Safe and Secure in Your Salesforce Enterprise: A Practical Guide
Guide to implement AI securely in your Salesforce
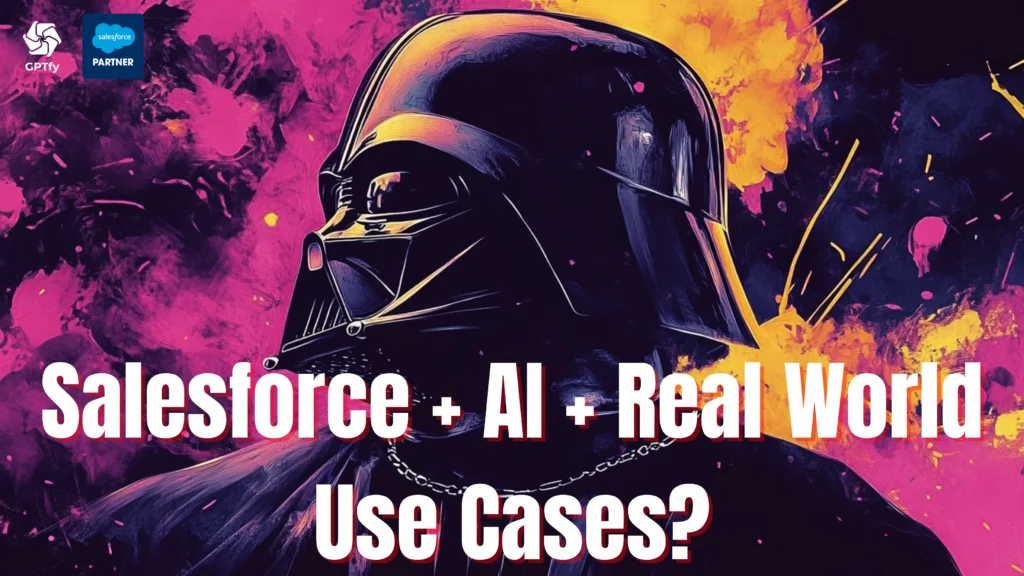
How Salesforce + AI Can Drive Real Business Value in Your Enterprise
Find out how you can improve your business with AI in Salesforce.
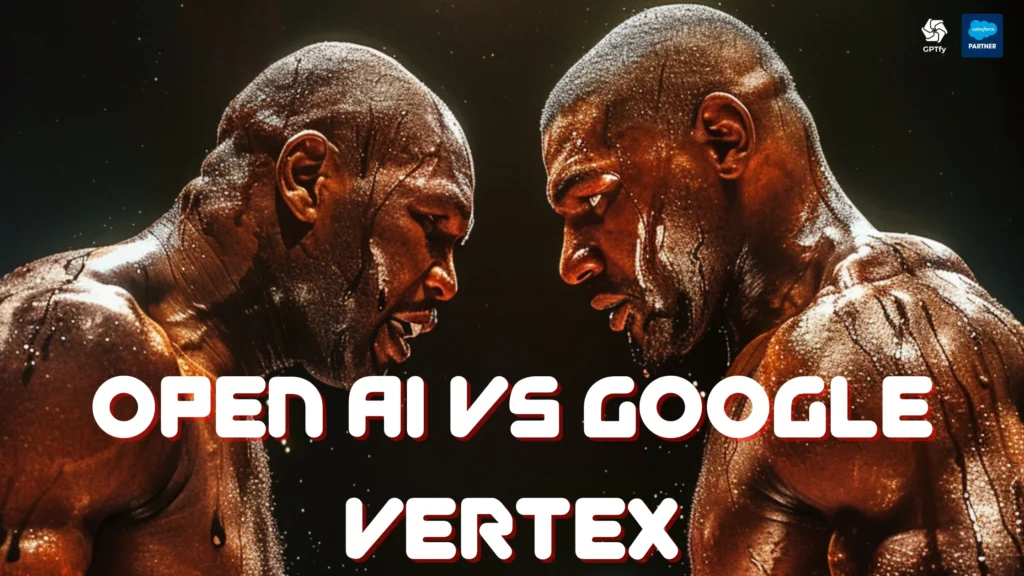
Open AI or Google Vertex Which is better for your AI + Salesforce
Find out what AI model makes sense for your Salesforce?
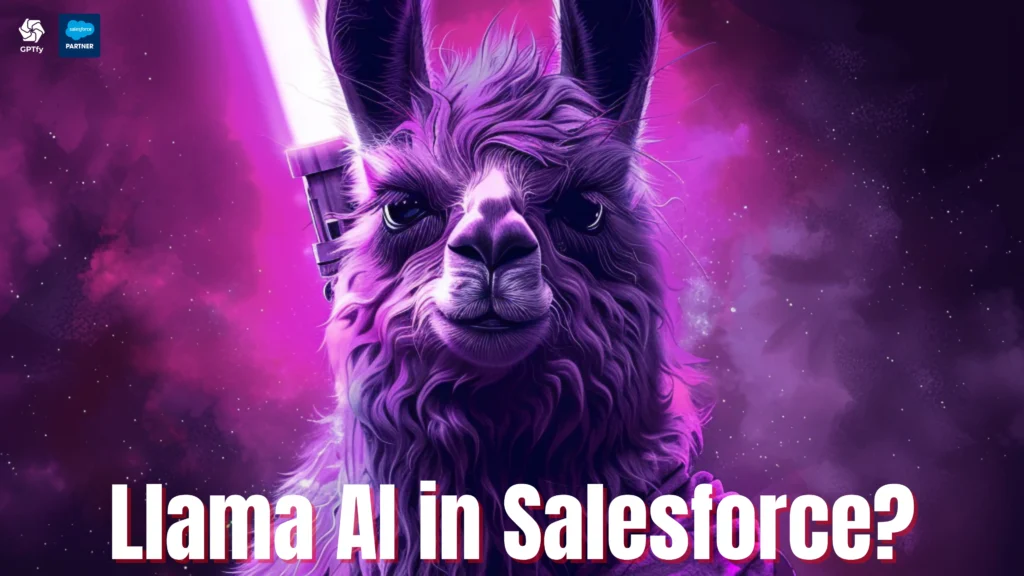
How to Bring Llama AI into Your Salesforce
Connect Llama, Meta’s Open-source AI model, to your Salesforce with GPTfy